Rock-Scissors-Paper
Two-in-One Logic Project
This project is really two projects. You build your own Rock-Scissors-Paper game in two different ways: (1) as an electric circuit board game, and/or (2) as a computer game. You can do either or both. If you do both you can compare your electric circuit logic to your computer program logic.
Rules of the game: Two players simultaneously choose either rock, scissors, or paper. The winner: scissors cut paper, paper wraps rock, rock smashes scissors.
Rock-Scissors-Paper
Electric Circuit Logic Project
How to play: Players A and B will simultaneously press their Rock, Scissors, or Paper buttons, and a lamp will indicate the winner.
Supplies you will need: A board, twelve switches (push-button spring-return normally-off micro-switches), two lamps (small bulbs or LEDs, and lamp sockets), batteries (and battery holders), wire, wire connectors, screws, tape, drill, screwdriver. Use twelve single-pole switches rather than six double-pole switches because that better demonstates how the circuit works.
How to make it: Mount everything on the board. If you want it to be pretty, put the lights and switches on the front and the wires and batteries on the back.
Incomplete Circuit Diagram
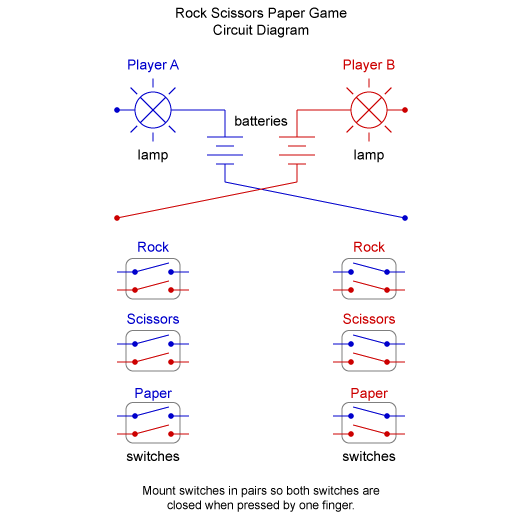
Before looking at the complete circuit diagram, see if you can figure out where the other wires go.
Complete Circuit Diagram
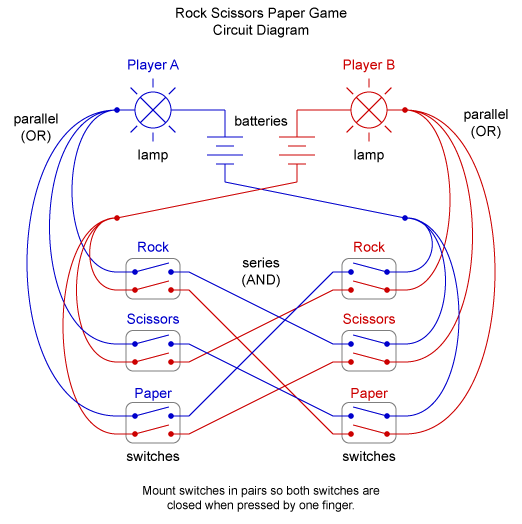
How it works: Each player has three ways to win. Player A's three ways to win are built from three circuits (blue), each of which has two switches, one for each player's choice, wired in series. The three series circuits representing the three ways for player A to win are wired in parallel to light up player A's lamp. Similarly for player B (red).
Teacher's note: Depending on the age of the students, you should let them figure out as much as they are able by themselves. Don't show them the complete circuit diagram. You may want to print the incomplete circuit diagram and draw in a few more wires.
Rock-Scissors-Paper
Computer Program Logic Project
How to play: This is a non-graphical command-line game. The program will ask player A to enter "r" (rock), "s" (scissors), or "p" (paper) (without letting player B see), then it will ask player B to enter "r", "s", or "p". The program will figure out who won.
Supplies you will need: A computer and the programming language software of your choice.
Incomplete Program
Here's the incomplete program in pseudo-code (not a real programming language).
write "Rock-Scissors-Paper Game" loop forever { write "Player A, enter r, s, or p: " read a (see note 1) write "Player B, enter r, s, or p: " read b write "A chose: " + a write "B chose: " + b if (...you figure out what goes here...) { write "A wins" } else if (...you figure out what goes here...) { write "B wins" } else { write "nobody wins" } }
Before looking at the complete program, see if you can figure out the logic to decide who wins. Use the boolean logic operators "AND" and "OR" with nested parentheses.
Note 1: You don't want player B to see what player A has typed, so you should (1) read without echoing what is typed, or (2) clear the screen after player A has typed, or (3) use a popup prompt box that goes away after player A has typed.
Complete Program
Here's the complete program in pseudo-code (not a real programming language).
write "Rock-Scissors-Paper Game" loop forever { write "Player A, enter r, s, or p: " read a (see note 1) write "Player B, enter r, s, or p: " read b write "A chose: " + a write "B chose: " + b if ((a="r" and b="s") or (a="s" and b="p") or (a="p" and b="r")) { write "A wins" } else if ((b="r" and a="s") or (b="s" and a="p") or (b="p" and a="r")) { write "B wins" } else { write "nobody wins" } }
Comparison of the Two Projects
Comparing the electric circuit to the computer program, notice how the series circuits correspond to the "AND" operators, and the parallel circuits correspond to the "OR" operators.